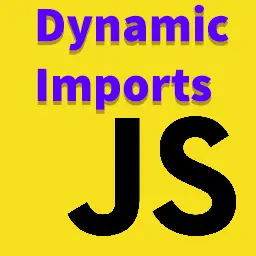
Dynamic Imports gotchas
Here’s a gotcha I ran into using dynamic imports with webpack.
This is valid:
import('module.js')
.then((module) => {
...
})
.catch(err => <loading error, e.g. if no such module>)
However this is not:
import(foo) // foo is variable module path
.then((module) => {
...
})
.catch(err => <loading error, e.g. if no such module>)
The gotcha:
It is not possible to use a fully dynamgsapic import statement, such as import(foo)
. Because foo
could potentially be any path to any file in your system or project.
The import expression must be given at least a hint of where the module is located. The reason is that bundling will be triggered for all potential files that could be import dynamically. For example import(`src/lib/${module}.js`)
will cause every *.js
files under 'src/lib/'
to be compiled. So be cautious of where you want to store your dynamic modules for importing.
After the promise resolved, you access your default export with module.default
. So for example if you export default class Search {}
, to create an object of Search, you would do new module.default()
You can also use Magic Comment for hinting:
import(
/* webpackPrefetch: true */
/* webpackPreload: true */
`./modules/${module}`
)
Note:
Although import()
looks like a function call, it’s a special syntax that just happens to use parentheses (similar to super()
).
So you can’t assign import
to a variable or use call/apply
with it. It’s not a function.